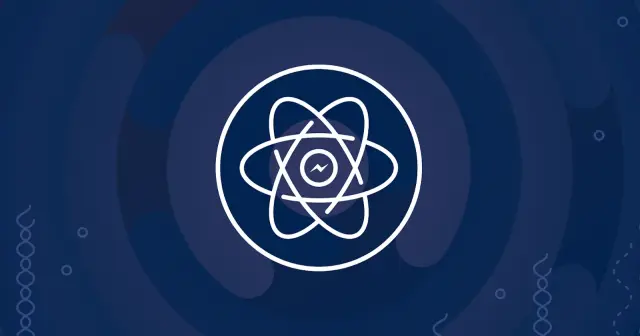
A First Look at React 18 with Vite and Netlify
Published:
Learn how to create a boilerplate React app with Vite, upgrade the application to React 18, and deploy the app to Netlify.
Outline
All of this project’s code can be found in the First Look monorepo on my GitHub.
Introduction
When it’s released, React 18 will include out-of-the-box improvements including:
- Automatic batching
- New APIs like
startTransition
- A new streaming server renderer with built-in support for
React.lazy
The React team has also taken a new step by creating the React 18 Working Group to provide feedback, ask questions, and collaborate on the release. The Working Group is hosted on GitHub Discussions and is available for the public to read.
React 18 Working Group
Members of the working group can leave feedback, ask questions, and share ideas. The core team will also use the discussions repo to share their research findings. As the stable release gets closer, any important information will also be posted on the React blog.
Because an initial surge of interest in the Working Group is expected, only invited members will be allowed to create or comment on threads. However, the threads are fully visible to the public, so everyone has access to the same information. The team believes this is a good compromise between creating a productive environment for working group members, while maintaining transparency with the wider community.
No specific release date is scheduled, but the team expects it will take several months of feedback and iteration before React 18 is ready for most production applications.
- Library Alpha: Available today
- Public Beta: At least several months
- Release Candidate (RC): At least several weeks after Beta
- General Availability: At least several weeks after RC
More details about the projected release timeline are available in the Working Group.
Create React App with Vite React Template
yarn create vite ajcwebdev-react18 --template react
Install Dependencies and Start Development Server
cd ajcwebdev-react18yarnyarn dev
If we look in our package.json
we’ll see React 18 is already included in the Vite template.
{ "name": "ajcwebdev-react18", "type": "module", "scripts": { "dev": "vite", "build": "vite build", "preview": "vite preview" }, "dependencies": { "react": "^18.2.0", "react-dom": "^18.2.0" }, "devDependencies": { "@types/react": "^18.0.28", "@types/react-dom": "^18.0.11", "@vitejs/plugin-react": "^4.0.0", "vite": "^4.3.2" }}
Use esbuild.jsxInject
to automatically inject JSX helper imports for every file transformed by ESBuild:
import { defineConfig } from 'vite'import reactRefresh from '@vitejs/plugin-react-refresh'
export default defineConfig({ plugins: [reactRefresh()], esbuild: { jsxInject: `import React from 'react'` }})
Root Component
import ReactDOM from 'react-dom'import './index.css'import App from './App'
const root = ReactDOM.createRoot( document.getElementById('root'))
root.render(<App />)
App Component
import logo from './logo.svg'import './App.css'
function App() { return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> <p>React 18 Deployed on Netlify with Vite</p> </header> </div> )}
export default App
Deploy to Netlify
echo > netlify.toml
[build] publish = "dist" command = "yarn build"
Initialize a Git repository and push to GitHub.
git initgit add .git commit -m "init"gh repo create ajcwebdev-react18 \ --public \ --push \ --source=. \ --description="An example React 18 application deployed to Netlify with Vite" \ --remote=upstream
Connect your GitHub repository to Netlify.
The build commands are included from your netlify.toml
.
Set a custom domain.
Go to your new domain (ajcwebdev-react18.netlify.app
in my case).