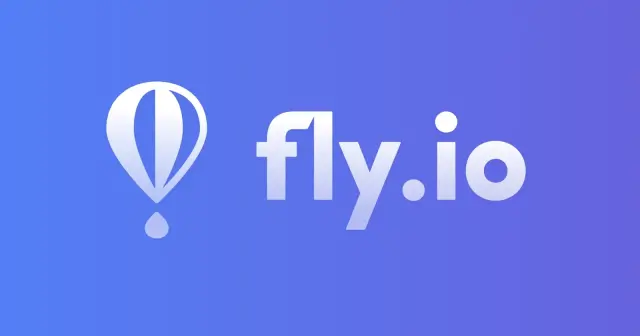
A First Look at Fly
Published:
Fly is a platform for fullstack applications and databases build with Dockerfiles or Buildpacks that need to run globally.
Outline
All of this project’s code can be found in the First Look monorepo on my GitHub.
Introduction
Fly is a platform for fullstack applications and databases that need to run globally. Fly executes your code close to users and scales compute in cities where your app is busiest. You can run arbitrary Docker containers and host popular databases like Postgres.
Fly Setup
Install flyctl
You can download the CLI on Mac, Linux, or Windows.
brew install superfly/tap/flyctl
Create Fly Account
If you are a new user you can create an account with flyctl auth signup
.
flyctl auth signup
After your browser opens you can either:
- Sign-up with your name, email and password.
- Sign-up with GitHub and check your email for link to set a password for verification.
You will also be prompted for credit card payment information, required for charges outside the free plan on Fly. See Pricing for more details.
Login to Fly Account
If you already have an account you can login with flyctl auth login
.
flyctl auth login
After your browser opens, sign in with your username and password. If you signed up with GitHub, use the Sign-in with GitHub button to sign in.
Create Project
mkdir ajcwebdev-flycd ajcwebdev-flynpm init -ynpm i express --saveecho > index.jsecho > Dockerfileecho > .dockerignoreecho 'node_modules\n.DS_Store' > .gitignore
Create Server
const express = require("express")const app = express()
const port = process.env.PORT || 3000
app.get("/", (req, res) => { greeting = "<h1>ajcwebdev-fly</h1>" res.send(greeting) })
app.listen(port, () => console.log(`Hello from port ${port}!`))
Run Server
node index.js
Hello from port 3000!
Create Dockerfile
Add the following code to Dockerfile
in the root directory of your project.
FROM node:14-alpineWORKDIR /usr/src/appCOPY package*.json ./RUN npm iCOPY . ./EXPOSE 8080CMD [ "node", "index.js" ]
Add the following code to .dockerignore
in the same directory as your Dockerfile
.
node_modulesDockerfile.dockerignore.git.gitignorenpm-debug.log
Launch Application on Fly
Run flyctl launch
in the directory with your source code to configure your app for deployment. This will create and configure a fly app by inspecting your source code and prompting you to deploy.
flyctl launch --name ajcwebdev-fly
Creating app in /Users/ajcwebdev/ajcwebdev-flyScanning source codeDetected Dockerfile appAutomatically selected personal organization: Anthony CampoloCreated app ajcwebdev-fly in organization personalWrote config file fly.tomlYour app is ready. Deploy with `flyctl deploy`? Would you like to deploy now? No
This creates a fly.toml
file.
app = "ajcwebdev-fly"
kill_signal = "SIGINT"kill_timeout = 5
[env]
[experimental] allowed_public_ports = [] auto_rollback = true
[[services]] http_checks = [] internal_port = 8080 protocol = "tcp" script_checks = []
[services.concurrency] hard_limit = 25 soft_limit = 20 type = "connections"
[[services.ports]] handlers = ["http"] port = 80
[[services.ports]] handlers = ["tls", "http"] port = 443
[[services.tcp_checks]] grace_period = "1s" interval = "15s" restart_limit = 6 timeout = "2s"
Add the following PORT
number under env
.
[env] PORT = 8080
Deploy Application
flyctl deploy
Show Current Application Status
Status includes application details, tasks, most recent deployment details and in which regions it is currently allocated.
flyctl status
App Name = ajcwebdev-fly Owner = personal Version = 0 Status = running Hostname = ajcwebdev-fly.fly.dev
Deployment Status ID = d94d886a-f338-28cf-4078-1ed838eea224 Version = v0 Status = successful Description = Deployment completed successfully Instances = 1 desired, 1 placed, 1 healthy, 0 unhealthy
InstancesID VERSION REGION DESIRED STATUS HEALTH CHECKS RESTARTS CREATED40591407 0 sjc run running 1 total, 1 passing 0 37s ago
Open your browser to current deployed application with flyctl open
.
flyctl open
Opening http://ajcwebdev-fly.fly.dev/
Visit your link (ajcwebdev-fly.fly.dev
in my case) to see the site.