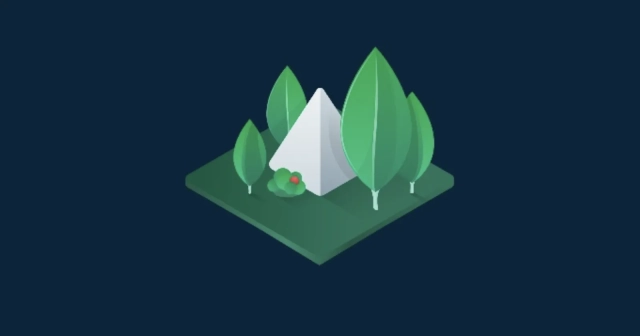
Can I Use MongoDB with Prisma Yet?
Published:
MongoDB is a database. It does stuff with data and then puts it in a base. Prisma now lets you do that without writing MongoDB stuff.
Outline
All of this project’s code can be found in the First Look monorepo on my GitHub.
Introduction
Warning: The MongoDB connector for Prisma 2 is not ready for production and should not be used by anyone under any circumstances. It is in Preview mode as of v2.27.0.
MongoDB is a database. It does stuff with data and then puts it in a base¹. But it doesn’t do this the way a normal database does. That’s because it uses documents instead of relations. At one point in time it was called NoSQL.
But don’t worry about all that.
The whole reason you use Prisma in the first place is because you don’t want to write SQL or whatever crazy query language they came up with at 10gen back in the day. You just want to write JavaScript like God intended.
And you’re willing to write lots and lots and lots and lots and lots and lots and lots of comments on GitHub letting the world know this.
Well it’s your lucky day…
Deploy Database on MongoDB Atlas
The first thing you’ll need is a MongoDB database (shocking). The easiest (not cheapest) way to get a MongoDB database is with MongoDB Atlas. I apologize for the click-ops. After creating an account you will be asked to name your organization and project.
You will then be asked for your preferred language, a question that no one has ever argued about.
You will then be asked if you want a plan that costs money or a plan that doesn’t cost money. I’m sure this will be a very hard decision to make.
Pick a region close to you cause ain’t nobody got time for the speed of light.
Again, you can pick the one that’s free or one that’s not free.
Give your cluster a name that suitably explains just how thoroughly this project has been nailed.
Click “Create Cluster” to create a cluster. You will then be taken to your dashboard.
Take a few minutes to contemplate your mortality. Once the existential dread starts to fade away you should have a cluster up and running.
If you want to do anything useful with this database like put data in the base then you need to connect to it. Click connect to connect.
Give your database a username and a password that is totally secret and not published in a blog post on the internet. Click “Create Database User” to create a database user and then click “Add your current IP address” to add your current IP address.
Click “Choose a connection method” to choose a connection method.
Click “Connect your application” to connect your application.
Create Prisma Project
Now that the boring stuff is out of the way we can get to the ORMing. Create a new project and initialize a package.json
.
mkdir ajcwebdev-prismongocd ajcwebdev-prismongonpm init -y
Install Dependencies
npm install -D prisma typescript ts-node @types/node
Initialize Prisma Schema
npx prisma init
If you’d like to try MongoDB on an existing Prisma project, you’ll need to make sure you’re running Prisma 2.21.2 or above. Just remember, no one under any circumstances should be using this. Not even you!!!
Prisma Schema
Your schema.prisma
file will contain a Post
.
datasource db { provider = "mongodb" url = env("DATABASE_URL")}
generator client { provider = "prisma-client-js" previewFeatures = ["mongodb"]}
model Post { id String @id @default(dbgenerated()) @map("_id") @db.ObjectId slug String @unique title String body String}
What’s that? You don’t need a blog? I don’t see what that has to do with anything.
Generate Prisma Client
Now run that one command that does all the stuff. Don’t worry about it too much, it just does the stuff.
npx prisma generate
Use Prisma Client
Create a script to test that we can read and write data to our base in an index.ts
file.
touch index.ts
import { PrismaClient } from "@prisma/client"const prisma = new PrismaClient()
async function main() { await prisma.$connect()
await prisma.post.create({ data: { title: "If you really want something, just complain loudly on GitHub", slug: "this-is-a-terrible-lesson-to-teach", body: "That's a joke please don't do this.", }, })
await prisma.post.create({ data: { title: "Second post", slug: "post-two", body: "This is the second post.", }, })
const posts = await prisma.post.findMany()
console.dir(posts, { depth: Infinity })}
main() .catch(console.error) .finally(() => prisma.$disconnect())
Environment Variable
Inside .env
include your DATABASE_URL
.
DATABASE_URL=mongodb+srv://ajcwebdev:dont-steal-my-db-ill-kill-you@nailed-it.5mngs.mongodb.net/myFirstDatabase?retryWrites=true&w=majority
Run Script
npx ts-node index.ts
If you followed along correctly you should get the following output:
[ { id: '6080d8df0011018100a0e674', slug: 'this-is-a-terrible-lesson-to-teach', title: 'If you really want something, just complain loudly on GitHub', body: "That's a joke please don't do this." }, { id: '6080d8e00011018100a0e675', slug: 'post-two', title: 'Second post', body: 'This is the second post.' }]
Next Steps
If you want to report an issue or file a feature request, please open an issue on GitHub. Since this is a preview feature that should not be used by anyone ever you may want to check open issues for known limitations.
[1] - Not an accurate definition of a database.